You will need to query using at-least one deploymentVar
as a filter. Hence you will need to deploy prompt chain versions before querying
them.
Prompt chains
Integrating SDK into your code
getPromptChain
function will respond with deployed version of the given prompt chain. You can deploy prompt chains directly from the Maxim dashboard.
For a prompt chain with specific deployment variables
For building query to get prompt chain with specific deployment variables, you can use QueryBuilder
.
Adding multiple queries
Querying Prompts
Sometimes you have usescases where you need to fetch multiple deployed prompt chains at once using a single query. For example, you might want to fetch all prompts for a specific customer or specific workflow. You can use getPromptChains
function for this purpose.
Query deployed prompt chains using folder
To get all prompts from a folder, you can use getPromptChains
function with folderId
as a query parameter.
First capture folder id
There are multiple ways to capture folder id. You can use Maxim dashboard to get folder id.
- Right click/click on three dots on the folder you want to get id for.
- Select
Edit Folder
option. - You will see folder id in the form.
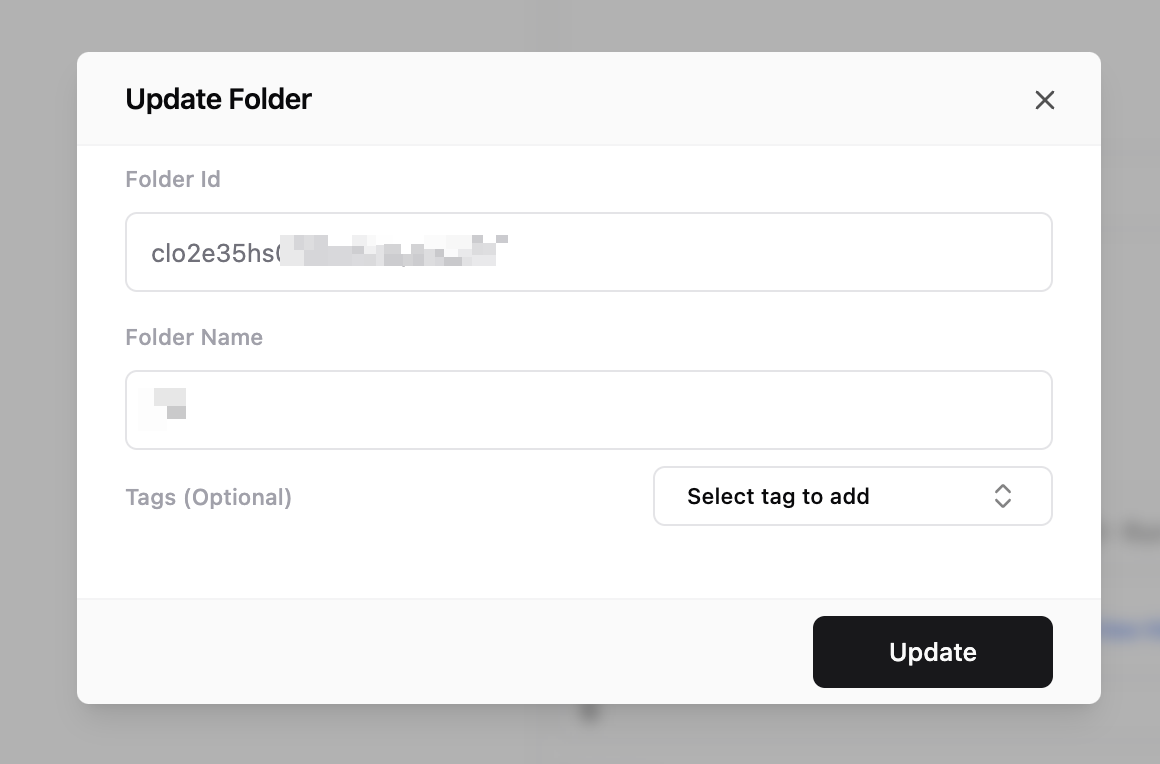
To get folders using tags attached to the folder.
Get all deployed prompts from a folder
You can filter prompt chains based on deploymentVars.
folder()
.Prompt chain Structure
Folder Structure
Using your own cache for prompts
Maxim SDK uses in-memory caching by default. You can use your own caching implementation by passing a custom cache object to the SDK. This allows you to remove complete dependency on our backend.
Interface for custom cache
You will have to pass this custom cache object to the SDK while initializing it.
Example
Here is the default in-memory cache implementation used by the SDK.
Matching algorithm
Before going into the details of how to use the SDK, let's understand how the matching algorithm works. Maxim SDK uses best matching entity algorithm.
- Let's assume that, you have asked for a prompt chain with deployment var
env
asprod
,customerId
as"123"
and a tag,tenantId
as456
forpromptId
-"abc"
. - SDK will first try to find a prompt chain matching all conditions.
- If we don't find any matching entity, we enforce only
deploymentVar
conditions (you can override this behaviour, as explained in the next section) and match as many tags as possible. - If we still don't find any prompt chain, we check for a prompt chain version marked as fallback.
- If we still don't find any prompt chain, we return
null
.
Overriding fallback algorithm
- You can override fallback algorithm by calling
.exactMatch()
onQueryBuilder
object. That will enforce all conditions to be matched.
- You can override fallback algorithm at each variable level. The third optional parameter in
deploymentVar
&tag
function isenforce
. If you passtrue
to this parameter, it will enforce exact match for that variable.