The CSVFile
class automatically validates your CSV headers against the data structure and provides type-safe access to your data.
Trigger Test Runs using SDK
Learn how to programmatically trigger test runs using Maxim's SDK with custom datasets, flexible output functions, and evaluations for your AI applications.
While Maxim's web interface provides a powerful way to run tests, the SDK offers even more flexibility and control. With the SDK, you can:
- Use custom datasets directly from your code
- Control how outputs are generated
- Integrate testing into your CI/CD pipeline
- Get real-time feedback on test progress
- Handle errors programmatically
Example of triggering test runs using the SDK
The SDK uses a builder pattern to configure and run tests. Follow this example to trigger test runs:
Copy your workspace ID from the workspace switcher in the left topbar
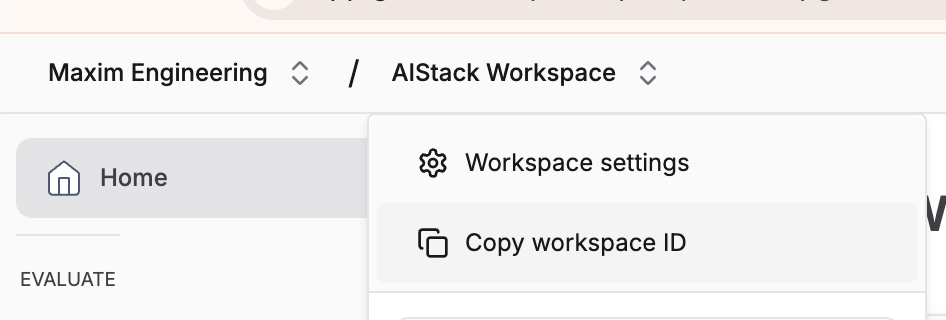
Understanding the data structure
Understand the data structure to maintain type safety and validate data columns. It maps your data columns to specific types that Maxim understands.
Basic structure
Define your data structure using an object that maps column names to specific types.
Available types
INPUT
- Main input text (only one allowed)EXPECTED_OUTPUT
- Expected response (only one allowed)CONTEXT_TO_EVALUATE
- Context for evaluation (only one allowed)VARIABLE
- Additional data columns (multiple allowed)NULLABLE_VARIABLE
- Optional data columns (multiple allowed)
Example
Working with data sources
Maxim's SDK supports multiple ways to provide test data:
1. Callable
2. Manual data array
For smaller datasets or programmatically generated data:
3. Platform datasets
Use existing datasets from your Maxim workspace:
Trigger a test on a workflow stored on Maxim platform
Find the workflow ID in the workflows tab and from menu click on copy ID.

Trigger a test on a prompt version stored on Maxim platform
To get prompt version ID, go to prompts tab, select the version you want to run tests on and from menu click on copy version id.

Custom output function
The output function is where you define how to generate responses for your test cases:
If your output function throws an error, the entry will be marked as failed and you'll receive the index in the failed_entry_indices
array after the run completes.
Adding evaluators
Choose which evaluators to use for your test run:
Human evaluation
For evaluators that require human input, setting up the human evaluation configuration is required and can be done as follows:
Custom evaluators
You can create custom evaluators to implement specific evaluation logic for your test runs:
Using custom evaluators
Once created, custom evaluators can be used alongside built-in evaluators:
Advanced configuration
Concurrency control
Manage how many entries are processed in parallel:
Timeout configuration
Set custom timeout for long-running tests:
Complete example
Here's a complete example combining all the features:
Scheduled test runs
Learn how to schedule test runs for your prompts, prompt chains and workflows at a regular interval.
Re-use your test configurations using presets
As your team starts running tests regularly on your entities, make it simple and quick to configure tests and see results. Test presets are a way to help you reuse your configurations with a single click, reducing the time it takes to start a run. You can create labeled presets combining a dataset and evaluators and use them with any entity you want to test.